Efficient and Readable Code
The importance of efficient and readable code (and low code configurations as well) cannot be overstated. It is the cornerstone of maintainable and scalable software. Clear and comprehensible code streamlines debugging and enhancements, and fosters collaboration among developers.
Handling Sensitive Data and Configurations using Variables
Embedding sensitive data such as links, passwords, and usernames directly into your code is not recommended. This practice can lead to security vulnerabilities and maintenance challenges. For example, if the path to a context on a distributed server changes, updating every hard-coded instance can be error-prone and time-consuming. The following is an example of bad practice, with hard coded values for a function:
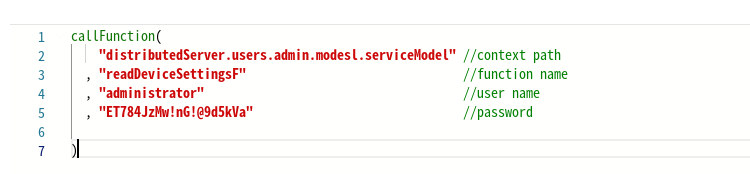
To enhance maintainability and security, store such information in variables. Take the case of the context path on a distributed server: by assigning it to a variable, any future changes require only a single update, ensuring consistency and reducing the risk of errors across the application.
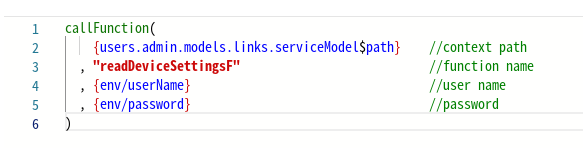
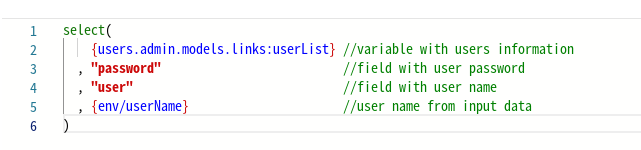
Deleting Commented Pieces of Code from Expressions
To debug expressions, it is sometimes necessary to replace environment variables with direct references. Since the debugging process may take several iterations, developers often use two or more versions of the code simultaneously; one of which is active and the remaining are enclosed in comment blocks. Be sure to remove such commented pieces of code after debugging to avoid application vulnerability, especially if the commented block contains confidential data like user names, passwords, and so on. The image below indicates a commented block that must be removed once debugging is completed:
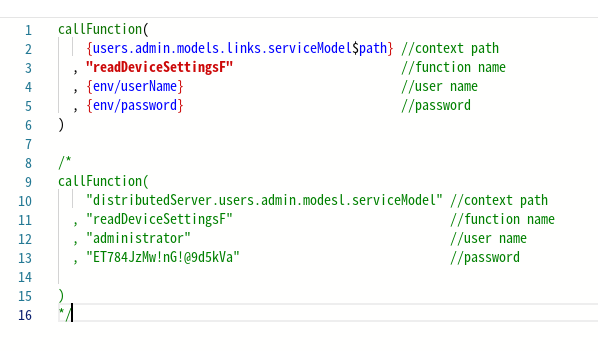
Using Tabs and Line Breaks for Nested Functions
Using tabs and line breaks is important for clarity in nested functions and argument lists when writing expressions. The code can be easier to understand when tabs and line breaks are used to delineate the layers of nesting, function arguments, and so on.
Expressions often contain several functions with multiple arguments. Writing all the arguments in one line without clear separation makes the expression cumbersome:

At first glance, it is difficult to understand which functions and arguments are being used in the expression, but by judiciously using tabulation and line breaks for every nested function, the code can be rewritten in a way that is easier to understand:
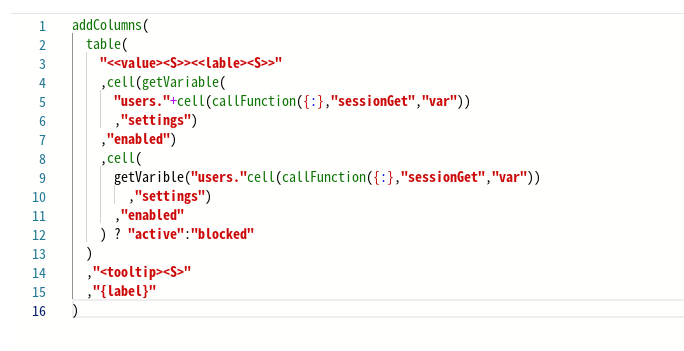
Placing Function Arguments in a Column
Most functions in the expression language require arguments. The description of each function contains possible arguments written in a single line, comma separated. If there are three or fewer arguments and each is relatively short, it is possible to enumerate them in a single line of the expression code. However, specifying the arguments of a function in a column can make the function more readable and easier to maintain. Advantages include being able to quickly see the number of arguments, see where they start and stop, easily comment out some of the arguments for quick debugging, and add comments for each argument. By convention, commas "," and the string concatenation operator "+" are placed at the beginning of the line to indicate the start of new arguments or the continuation of a string from the previous line.
Here are some examples:
Suppose one table in the expression is based on another table. As a result there are many function arguments and listing them in one line will make the code difficult to read:

Listing the arguments in a column makes the code much more readable. The string defining the table is split into two lines using the concatenation operator “+”, and further arguments are each written on a separate line:
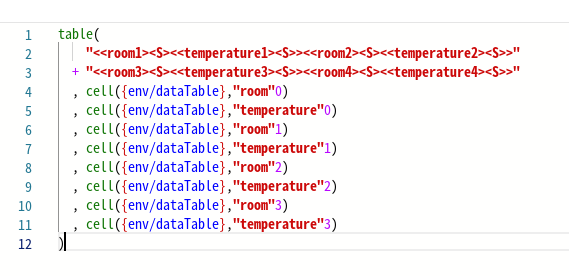
The comma character ",” at the beginning of the line helps define which and how many arguments are used in the function. During debugging, having each argument on a separate line allows an individual argument to be commented out, if needed, or for different values to be easily inserted into the function arguments:
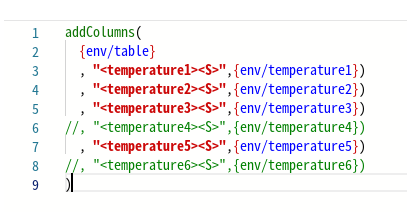
Split Long String Rows
Arguments of many functions are specified as strings in the expression language. Arguments with long strings can make expressions difficult to read. In such cases, splitting a long string into several shorter ones can simplify writing, debugging, and maintaining expressions. To split a string expression, put a closing quote in the appropriate place, use the concatenation operator "+" and an opening quote before the rest of the text. This method is very convenient for splitting function arguments that contain long strings. Take, for example, a function with a long string:
exampleFunction("suppose this is a very long string, that just goes on and on and on, no one knows where it stops, unless they scroll to the end of the line.")
The preceding function can be split into multiple lines as follows:
exampleFunction(
"suppose this is a very long string, that just goes on and on and on, "
+ "no one knows where it stops, unless they scroll to the end of the line."
)
The following example illustrates string splitting while working with SQL query. A query can refer to a set of contexts, data tables, variables, and more. Placing them in one line will make the query cumbersome. Splitting the long query string into several shorter ones will make the code more readable and simplify further maintenance and debugging. Placing each variable on a new line allow you to quickly comment, add, remove or replace any line accurately:
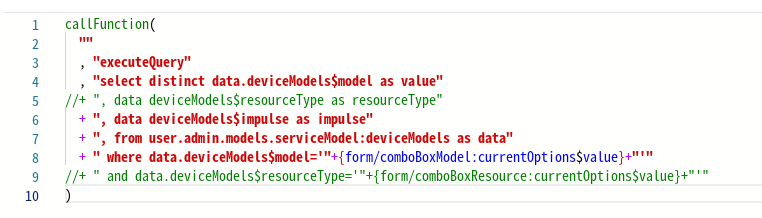
Use Single Quotes for Outer Strings and Double Quotes for Inner Strings
When writing expressions, it is a common convention to use single quotes for the outermost strings and double quotes for strings nested within. This approach simplifies function nesting and minimizes quote conflicts.
Adopting single quotes for the outer layer and double quotes for inner strings ensures that the inner string is interpreted literally, without being processed as part of the code that surrounds it. This practice also makes it easier to include functions or expressions without the complication of escaping quotes.
Here's an example demonstrating the use of single and double quotes:
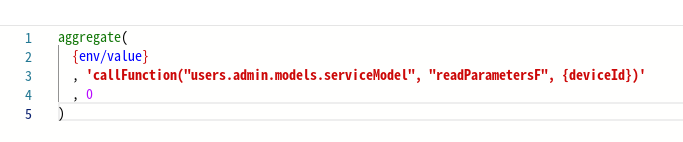
Decomposing Complex Expressions
Sometimes tabulation and line breaks are not enough to give clarity, especially with expressions that contain numerous functions, with nested and sequential operations. At a certain level of complexity, consider using breaking up a single complex expression into a rule set inside a model. A complex expression can be decomposed into simpler parts, which in turn simplifies both the development and maintenance of the desired functionality.
Example Decomposition
Suppose a function implementation implies several sequential actions. If we write the function in a single line, the expression will be as follows, and quite difficult to be understood by a human:

Even introducing multiple lines and using tabulation to show hierarchy, this expression is quite cumbersome. In this case, the expression can be decomposed into separate parts and expressed as a ruleset. As shown below, each part of the expression has been separated into a step in a rule set, with each step clearly commented with a description of its purpose.
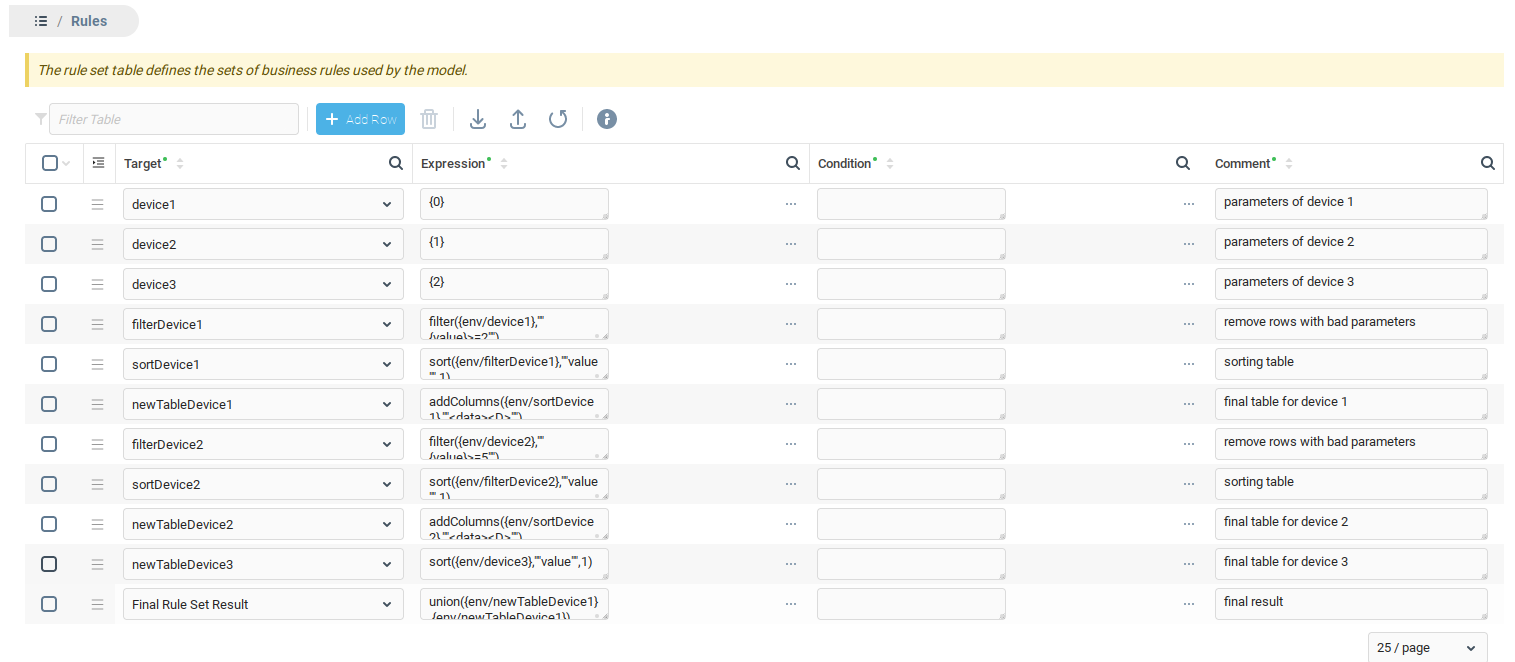
Was this page helpful?