General Best Practices
Some general practices that can help improve work processes, avoid common mistakes, and achieve better results. These tips cover a wide range of topics that can help keep your projects maintainable.
Choose A Single Language to be Used for All Non-Client Facing Text
In a work environment where engineers are communicating with each other in multiple languages, be sure to choose a single common language for all names and comments. This ensures that all engineers will be able to understand the purpose and functioning of the commented context.
Be Systematic in Avoiding Typographical Errors
Typos in contexts, function names, and variable names can have severe consequences, as they can affect multiple aspects of a project. Correcting such mistakes can be time-consuming and labor-intensive
Example of typos:
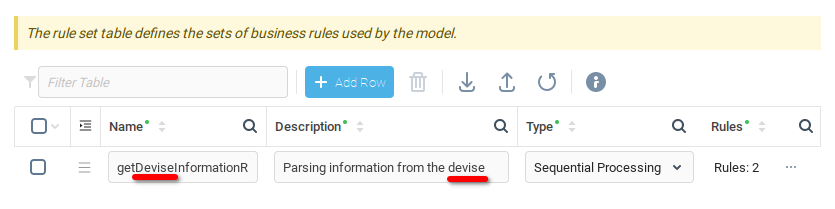
At the very least, this kind of mistake can make it harder to use context references. In the example above, an engineer will get an error trying to reference the rule with the name getDeviceInformation
, since the actual rule name contains a misspelling with the word “devise”.
Backup Your Work Regularly
Make sure to backup all the contexts or tables you work with (by enabling storage of change history for your resources)
When working on large projects with many other people, increase the frequency of backups to ensure that everyone's work is safely backed up
Create Events for Important Actions
A model can execute various processes. It is best practice to generate events after completing important actions, such as "start of synchronization" or "end of synchronization." However, events should be used judiciously, since triggering them too frequently can put an unnecessary load on the server.
If a device has a CRC (cyclic redundancy check) of its configuration, it should be checked at every synchronization. If the received CRC is incorrect, an event should be generated. This allows for a history of configuration changes to be tracked and alerts to be sent to the responsible parties.
If a function or ruleset requires time to execute, an event should be fired at its completion. This event can then serve as an activator for bindings that trigger further steps, ensuring that actions do not attempt to access data which has not been generated.
Fill in the "Description" and "Comments" Fields
Other users should be able to understand the purpose of a context, function, or other element from its description or comments.
Avoid using comments like "Don't touch it" or "I don't know how it works".
Adding comments for a step in a Rule Set will help you and other engineers understand the purpose of the step:
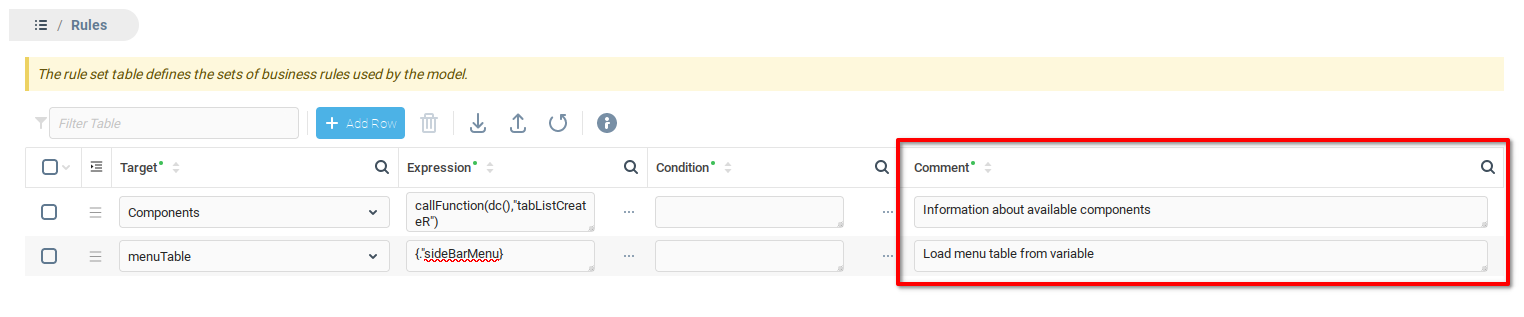
Use Validators to Ensure Data Cleanliness
To prevent incorrect or unexpected input from users, it is important to use validators any time data is being collected through an interface. To ensure that users understand the rules for filling in input data, consider including a tooltip or error message.
Regular expressions (regex) can be used as validators. Here are two examples:
For expressions in bindings for dashboards, use the following rule to allow any digit:
{env/value}~=([0-9]+)
.For variables, select the special option and choose the type, then write a rule. For example, to allow only lowercase Latin letters, use the following rule:
{env/value}~=([a-z]+)
.
Make sure to specify where the special option for variables can be found, such as in a dropdown menu or settings panel.

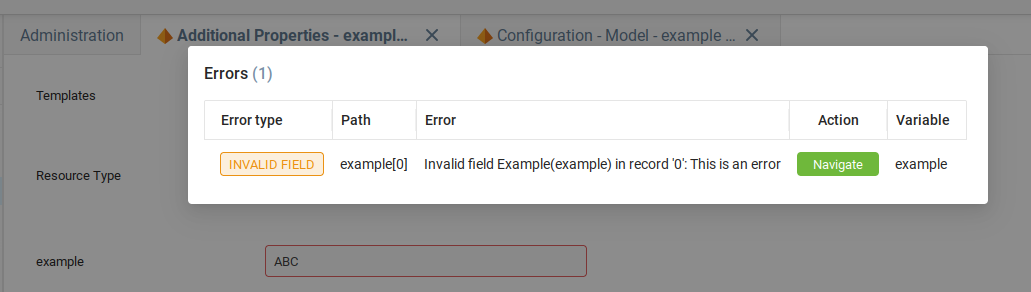
An example error during password validation:
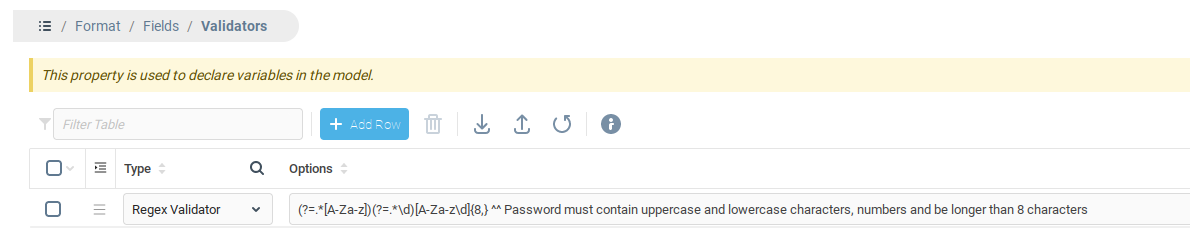
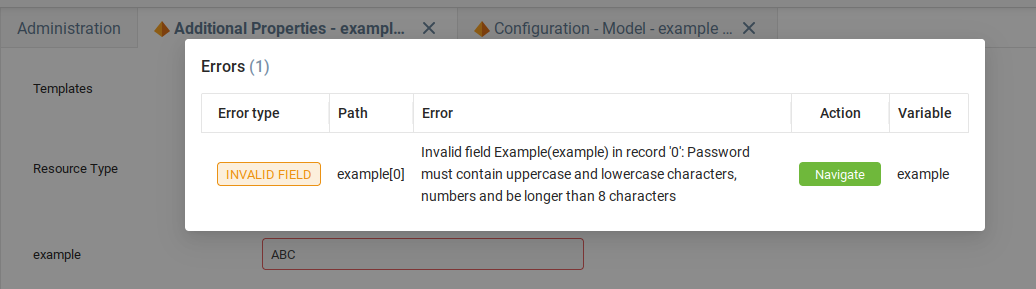
An example interface for entering a telephone number. The validation method enforces only digits, and displays a certain formatting to help the user understand it is a phone number, even if they do not read the label.
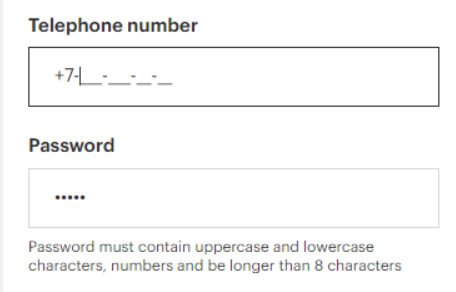
Use Java Instead of Expressions Only when Necessary
Not everyone may have proficiency in Java, which can create difficulties for other engineers working on or supporting the project. One solution is to determine useful "Java code" functions and add them to the base platform functionality, allowing all engineers to access these functions by triggering scripts with the expression language.
Some cases where it may be appropriate to create such scrips include:
When the
aggregate
andaddColumns
functions are not sufficient for a cycle: For example, if you need to add a nested function like "aggregate(aggregate,...),...,..." it is a good indicator to use a Java script. If additional nesting levels are required, it is highly recommended to use scripts.When working with large data arrays: For analyzing data arrays, scripts may be a better option. For example, a function for calculating CRC.
When using uncommon logic for string parsing: If the incoming string has a varying length and parsing parameters change position each time, a script may be a better solution.
See Best Practices for Writing Scripts to get more advice.
Avoid Using Periodical Bindings
Using periodical bindings can consume system resources, create spam in the event log, and can be difficult to maintain. Therefore, periodic bindings are generally considered inefficient practice. Intead, event-driven bindings should be used whenever possible.
If you still need to perform actions periodically, consider using scheduled jobs.
Group Dashboard Bindings in a Logical Order
Entries in the bindings table can be reordered with a drag-and-drop operation. Grouping related bindings together can make it easier for other engineers to understand how the project works. The examples below show how the engineer has created section breaks by adding bindings with empty targets and comments in the expression field. There are many valid organizing principles which could be applied when grouping bindings, what is most important is that all engineers in the organization follow the same organizing principle. See the examples of grouped bindings below.
Group bindings by visible area (steps in wizard, tabs from a tabPanel)
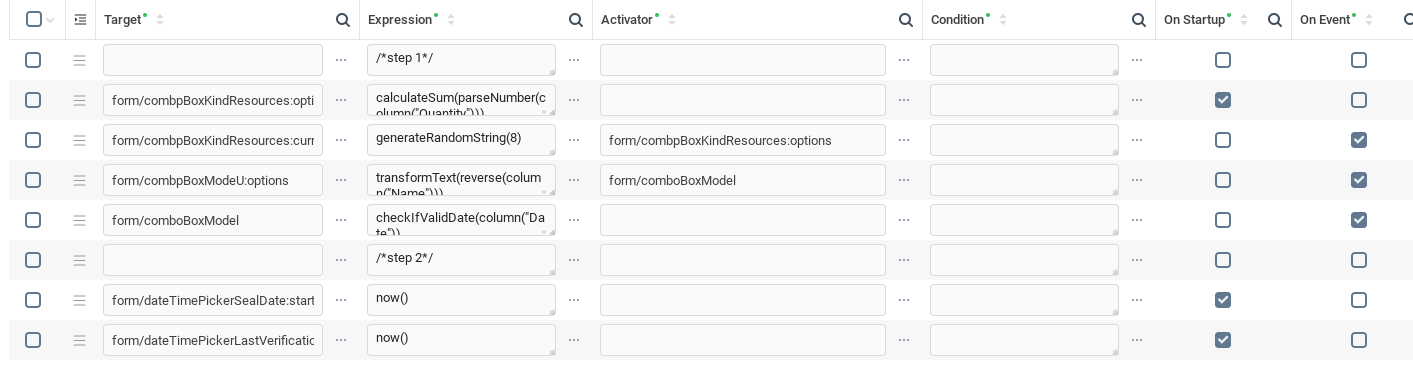
Locate related bindings one after another
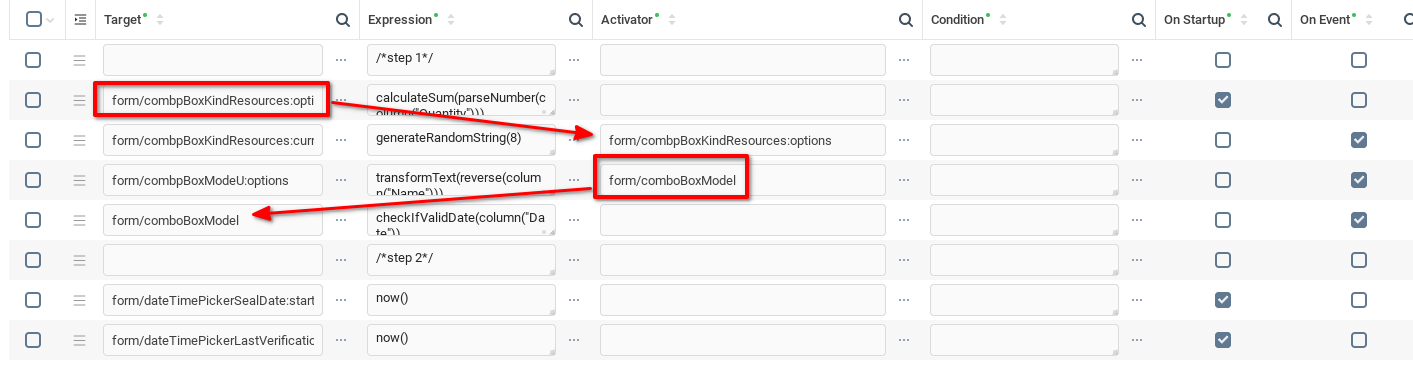
Suppose you have two-tabbed container, where the first tab contains a Dropdown List and a Data Table, while the second tab features a Line Chart. When a user selects an option from the Dropdown List, both the Data Table and the Line Chart should be updated.
There are two ways to achieve this. The bad implementation is to activate the binding for both charts every time the user selects an option from the Dropdown List, which wastes resources by activating the binding many times to update a chart which is not visible to the user.
A better approach is to activate the bindings only for the chart in the visible tab. Careful consideration of how the user interface is structured can lead to even more efficient binding activation strategies.
Make Use of Custom Properties as Transit Points Between Data from Back-End and Front-End.
Custom properties are useful in situations where the data for a given element depends on several other elements. Without a custom property to aggregate and validate the data, an error in a single element could cause the whole dashboard to crash. A typical scenario is to create a custom property with a table to serve as an intermediary data store for all elements. Working with a single table in custom properties and creating links to it is easier than creating links between many separate variables.
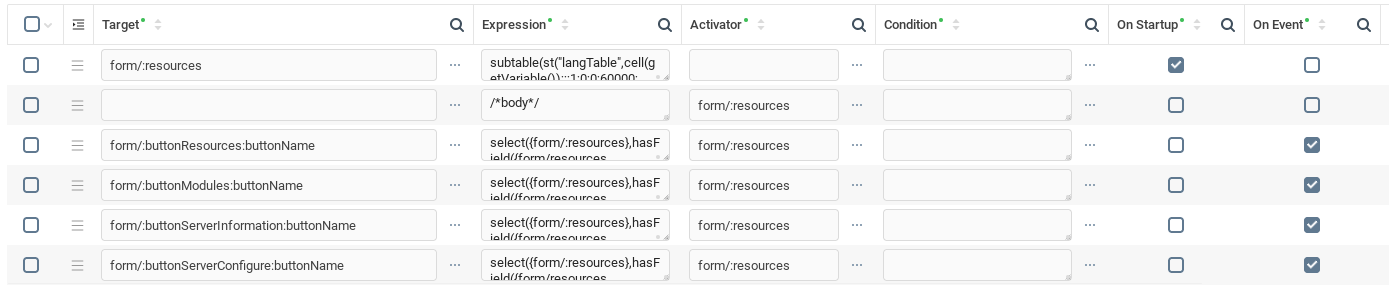
Regularly Refer to the Knowledge Base and Documentation
Documentation and the knowledge base serve as critical repositories of knowledge that can significantly enhance productivity and problem-solving within an organization. When faced with challenges or unfamiliar tasks, leverage both of these resources to find solutions or guidance.
Utilizing Documentation for Problem-Solving
When you encounter obstacles during your work, consider the following steps:
Identify the Issue: Clearly define what problem you are facing.
Consult the Documentation: Look for relevant sections within the documentation and knowledge base that address similar issues.
Apply Found Solutions: Use any applicable instructions or insights to resolve your issue.
Contributing to the Community
Beyond being a consumer of information, there is significant value in contributing back to the Iotellect user community:
Publish Your Insights: If you devise a new solution or discover a more efficient method, document it for others
Update Existing Material: Keep information current by revising outdated content when necessary
Encourage Collaboration: Foster an environment where colleagues are motivated to both use and contribute to documentation
Benefits of Active Participation
Active participation in creating and maintaining a knowledge base has several benefits:
It ensures that knowledge is preserved and easily accessible
It saves time for others who may face similar challenges by providing ready-made solutions
It promotes continuous learning and improvement within teams
Using the "Sleep" Function is (Almost Always) Bad Practice
The sleep
function is a programming construct that introduces a delay in program execution. It is commonly used to pause the flow of code, allowing for timed sequences or waiting periods between actions. While this can be useful in certain scenarios, it's important to understand its implications on system performance and user experience.
Potential Issues with Sleep Function
Relying on sleep
can create dependencies on system performance which may vary across different servers or after server upgrades. This variability can lead to two main issues:
Logic Breakdown: The timing logic may no longer hold true if system performance changes, potentially causing processes to fail.
User Experience: Users might experience unnecessary delays as they wait for actions to complete, especially if the sleep duration is not optimized.
Alternatives to Sleep: Event-Driven Activation
To avoid these pitfalls, it's advisable to use event-driven mechanisms that provide more control and reliability:
Event Firing: Implement events that trigger subsequent actions once certain conditions are met or tasks are completed.
Control Points: Create specific points within your code where control is passed from one action to another based on events rather than arbitrary time delays.
Appropriate Uses of the Sleep Function
Despite its limitations, there are cases where using sleep
makes sense, for example, Sequential Device Property Writing: When interacting with devices that require a set amount of time between writing properties, employing a sleep
function within a loop can ensure each property has sufficient time for processing before moving onto the next one.
Inappropriate Uses and Solutions: Dashboard Example
Consider a dashboard application with buttons triggering complex operations like context creation followed by screen transitions:
Inappropriate Use: Inserting
sleep
between context creation and screen transition could lead users into thinking the application has crashed due to unresponsive behavior.Solution - Keyword Activation: Instead of using sleep, you could implement keyword activation where you store results from one operation (context creation) into custom properties which then act as triggers for subsequent operations (screen transition).
Solution - Special Event Firing: Alternatively, firing special events at critical junctures allows you seamlessly chain dependent operations without arbitrary delays.
By adopting these strategies over indiscriminate use of sleep
, developers can build more robust applications that respond dynamically according their internal logic rather than relying on fixed-duration pauses which may not align well with varying system performances or user expectations.
Make Reports Fully Parameterized
To ensure the highest level of flexibility and maintainability in reporting, it is imperative that all parameters used within a report are fully parameterized. This practice enables users to adjust and control various aspects of the report seamlessly without having to directly modify the underlying report template itself.
Poor Practice Example:
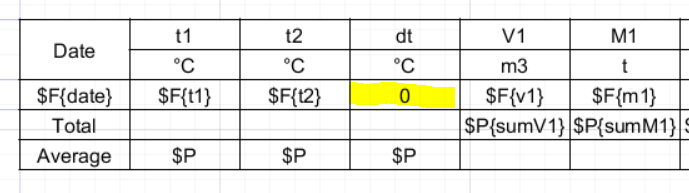
In this example, we observe that while most elements of the table have been parameterized, there is one cell—clearly highlighted—that has not been. The lack of parameterization in this cell means that any changes required for its content or data source will necessitate manual adjustments. This can lead to several issues:
Increased Risk of Errors: Manual changes are prone to human error, which can result in inaccuracies within the report.
Reduced Efficiency: Time must be spent locating and modifying this specific cell for each instance where a change is needed.
Lack of Scalability: As reports grow more complex, manually updating individual cells becomes increasingly impractical.
To avoid these pitfalls, it's crucial that every element within a report is designed with full parameterization from inception. By doing so, you ensure that updates or modifications can be made quickly through parameter adjustments rather than labor-intensive direct edits to the template or generated reports.
Was this page helpful?